VM-STUDYPOINT:
Complete Microsoft SDE Interview Preparation Guide—everything in one place.👇
🧠 1. Core Computer Science Concepts
✅ Operating Systems
-
Process vs Thread:
-
Process: Independent, separate memory space
-
Thread: Lightweight process, shares memory with other threads in same process
-
-
Deadlock: Circular wait for resources.
-
Prevent using: ordering of resource requests, timeout, deadlock detection
-
✅ DBMS
-
Normalization:
-
1NF: No repeating groups
-
2NF: No partial dependency
-
3NF: No transitive dependency
-
-
Transactions (ACID):
-
Atomicity, Consistency, Isolation, Durability
-
-
Indexing: Faster lookups (B-Tree, Hash-based)
✅ Computer Networks
-
TCP vs UDP:
-
TCP: Reliable, ordered (e.g. HTTP)
-
UDP: Fast, no guarantee (e.g. video stream)
-
-
DNS: Converts domain name to IP
-
OSI Model: 7 layers – Physical to Application
✅ OOP Concepts
-
Encapsulation: Hide internal state
-
Abstraction: Expose only essentials
-
Inheritance: Reuse code
-
Polymorphism: One interface, many forms (method overriding, overloading)
📊 2. DSA (Data Structures & Algorithms)
🔹 Must-Practice Topics:
-
Arrays, Strings
-
HashMaps, Sets
-
Linked Lists
-
Trees (Binary Tree, BST)
-
Graphs (BFS/DFS)
-
Recursion, Backtracking
-
Sliding Window, Two Pointer
-
Dynamic Programming
🔹 Examples in Python & C++
Q1: Two Sum (HashMap)
Python:
pythondef two_sum(nums, target):
map = {}
for i, num in enumerate(nums):
if target - num in map:
return [map[target - num], i]
map[num] = i
C++:
cppvector<int> twoSum(vector<int>& nums, int target) {
unordered_map<int, int> map;
for (int i = 0; i < nums.size(); ++i) {
if (map.count(target - nums[i]))
return {map[target - nums[i]], i};
map[nums[i]] = i;
}
return {};
}
Q2: Detect Cycle in Linked List (Floyd’s)
Python:
pythondef has_cycle(head):
slow = fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
if slow == fast:
return True
return False
Q3: Longest Substring Without Repeating Characters
Python (Sliding Window):
pythondef lengthOfLongestSubstring(s):
left, max_len = 0, 0
seen = {}
for right in range(len(s)):
if s[right] in seen:
left = max(left, seen[s[right]] + 1)
seen[s[right]] = right
max_len = max(max_len, right - left + 1)
return max_len
🏗️ 3. Basic System Design (For Freshers)
Q: Design a URL Shortener
-
Short Key =
Base62(id)
-
Store original ↔ short mapping in DB
-
Cache top links with Redis
-
REST API to create and redirect
-
Optional: Analytics (count, geo info)
Tips:
-
Know basic components (DB, cache, load balancer)
-
Mention horizontal scaling
-
No need for code unless asked
💬 4. Behavioral Questions (STAR Format)
Q: Tell me about a time you faced a technical challenge.
S: Our app crashed during login.
T: I had to debug and fix under pressure.
A: Used logging, binary search approach in code to isolate the bug.
R: Found the issue in token parsing and fixed it — prevented 20+ future crashes.
Q: Why Microsoft?
-
I'm passionate about products like VS Code, GitHub, and Azure.
-
Microsoft values innovation, inclusion, and global impact — aligns with my goals.
-
I want to grow in a place where I’m constantly learning from brilliant minds.
🧪 5. Microsoft Interview Process (Fresher - SDE)
Round | Details |
---|---|
OA | Codility/HackerRank: 2-3 DSA Qs (60-90 min) |
Tech 1 | DSA + OOPs + CS core |
Tech 2 | Projects, basic system design |
HR | STAR, resume deep-dive, teamwork, why MS |
🧠 6. Microsoft Online Assessment Tips
-
Use Python if you're comfortable — concise + fast to write
-
Practice on LeetCode, HackerRank, and InterviewBit
-
Use dry-run technique for debugging
-
Always communicate your thought process clearly
📆 7. 30-Day Study Plan
Week | Focus |
---|---|
1 | Arrays, Strings, Hashing, OS, DBMS |
2 | LinkedList, Trees, Sorting, OOPs |
3 | Recursion, Graphs, CN, System Design |
4 | Mock interviews, STAR prep, project revision |
🔗 Resources
Let's level up and dive into mock Microsoft interview questions section-wise, with full answers and explanations so you get the feel of the real thing. Let’s break this down into:
🔥 Section 1: Data Structures & Algorithms — Mock Interview Q&A
Q1. Merge Intervals
Given: A list of intervals
[[1,3],[2,6],[8,10],[15,18]]
Output:[[1,6],[8,10],[15,18]]
🧠 Idea: Sort by start time, merge overlapping ones.
Python:
pythondef merge(intervals):
intervals.sort()
merged = []
for i in intervals:
if not merged or merged[-1][1] < i[0]:
merged.append(i)
else:
merged[-1][1] = max(merged[-1][1], i[1])
return merged
👉 Microsoft Favorite for testing sorting logic + edge cases.
Q2. Find Median in a Data Stream
You’re given a stream of integers. Design a class to return the median at any time.
🧠 Use two heaps: max-heap (left half) & min-heap (right half)
Python (using heapq
)
pythonimport heapq
class MedianFinder:
def __init__(self):
self.low = [] # max heap (invert values)
self.high = [] # min heap
def addNum(self, num):
heapq.heappush(self.low, -num)
heapq.heappush(self.high, -heapq.heappop(self.low))
if len(self.low) < len(self.high):
heapq.heappush(self.low, -heapq.heappop(self.high))
def findMedian(self):
return (-self.low[0] + self.high[0]) / 2 if len(self.low) == len(self.high) else -self.low[0]
🧠 Microsoft asked this in SDE intern round (2023).
💻 Section 2: OOPs and System Design (Fresher Level)
Q3. Design a Parking Lot (Object-Oriented Design)
Requirements:
-
Support for multiple levels
-
Each level has slots for cars, bikes
-
Methods:
parkVehicle()
,leaveSlot()
,getFreeSlots()
🧱 Classes:
-
Vehicle
(base) -
Car
,Bike
(inherits Vehicle) -
Slot
,ParkingLot
,Level
What they look for:
-
Clean inheritance
-
Association (Lot has Levels, Levels have Slots)
-
Not code—just class diagram + logic
Q4. Design an Online Code Editor (like LeetCode)
Ask: What components would you use?
✔️ Components:
-
Frontend: Code editor (Monaco/ACE)
-
Backend:
-
Auth & DB
-
Code runner (sandboxed docker container)
-
REST APIs to fetch questions, run code
-
-
Database: Store submissions, questions, users
👉 Talk about modularity, microservices, and security in sandboxing
🗣️ Section 3: HR + STAR Questions
Q5. Tell me about a time you worked in a team to complete a project.
S: Final year group project to build an expense tracking app
T: I was responsible for backend APIs
A: Used Flask + SQLite, created modular code, helped frontend with API integration
R: We submitted early and demo’d to great feedback—team got best project award
✅ Keep answers within 90–120 seconds using STAR
Q6. How do you handle failure?
"I believe failure is a part of the learning process. In one hackathon, our app crashed midway. Instead of giving up, we debugged overnight and presented a partial version that still showed our concept. We didn’t win, but the experience taught me quick decision-making and resilience."
📈 Want More?
Here’s what I can add next for you:
-
More medium/hard DSA questions with step-by-step approach
-
Mock behavioral Qs tailored to your resume
-
Project-based questions: How to present your academic/final year projects smartly
-
Microsoft internship/graduate round insights from past candidates
-
Resume & LinkedIn optimization tips for Microsoft
Pages
Labels
- 4TH YEAR CSE
- 4th sem question paper
- 7th sem
- 8th sem
- BE
- Image processing
- MACHINE LEARNING AND AI
- MLAI
- MLANDAI
- Object Oriented Analysis and Design
- cse
- data warehouse and mining
- dwm
- engineering
- engineering study material
- imp
- ip
- notes
- objectorianted analysis and design
- ooad
- ssee
- study material
- study point
Search This Blog
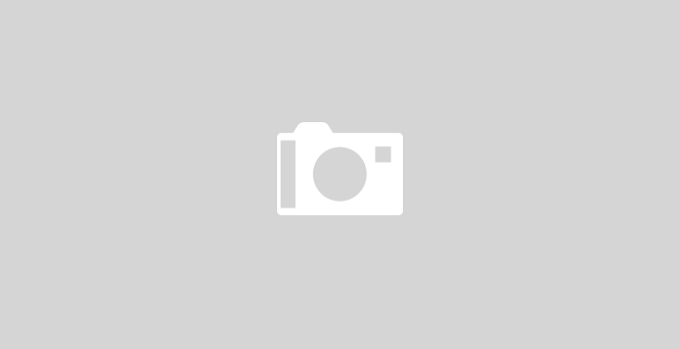
Social Plugin